C programming language multiple choice questions and answers with explanation for interview
What will be output if you will compile and execute the following c code?
void main(){
int i=320;
char *ptr=(char *)&i;
printf("%d",*ptr);
}What will be output if you will compile and execute the following c code?
#define x 5+2
void main(){
int i;
i=x*x*x;
printf("%d",i);
}
What will be output if you will compile and execute the following c code?
void main(){
char c=125;
c=c+10;
printf("%d",c);
}
Explanation:
As is well-known, the
char
data type exhibits cyclic properties. When char
variables are increased or decreased beyond their maximum or minimum values, respectively, they repeat the same value in accordance with the cyclic order as follows: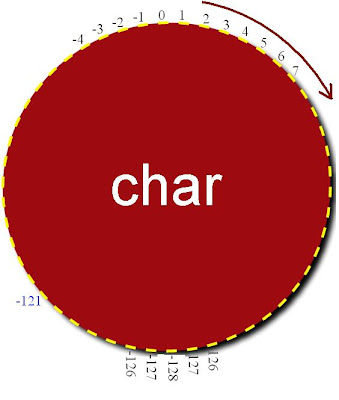
So,
125+1= 126
125+2= 127
125+3=-128
125+4=-127
125+5=-126
125+6=-125
125+7=-124
125+8=-123
125+9=-122
125+10=-121
What will be output if you will compile and execute the following c code?
void main(){
float a=5.2;
if(a==5.2)
printf("Equal");
else if(a<5.2)
printf("Less than");
else
printf("Greater than");
}
What will be output if you will compile and execute the following c code?
void main(){
int i=4,x;
x=++i + ++i + ++i;
printf("%d",x);
}
What will be output if you will compile and execute the following c code?
void main(){
int a=2;
if(a==2){
a=~a+2<<1;
printf("%d",a);
}
else{
break;
}
}
What will be output if you will compile and execute the following c code?
void main(){
int a=10;
printf("%d %d %d",a,a++,++a);
}
What will be output if you will compile and execute the following c code?
void main(){
char *str="Hello world";
printf("%d",printf("%s",str));
}
What will be output if you will compile and execute the following c code?
#include "stdio.h"
#include "string.h"
void main(){
char *str=NULL;
strcpy(str,"cquestionbank");
printf("%s",str);
}
What will be output if you will compile and execute the following c code?
#include "stdio.h"
#include "string.h"
void main(){
int i=0;
for(;i<=2;)
printf(" %d",++i);
}
What will be output if you will compile and execute the following c code?
void main(){
int x;
for(x=1;x<=5;x++);
printf("%d",x);
}
What will be output if you will compile and execute the following c code?
void main(){
printf("%d",sizeof(5.2));
}
What will be output if you will compile and execute the following c code?
#include "stdio.h"
#include "string.h"
void main(){
char c='\08';
printf("%d",c);
}
What will be output if you will compile and execute the following c code?
#define call(x,y) x##y
void main(){
int x=5,y=10,xy=20;
printf("%d",xy+call(x,y));
}
What will be output if you will compile and execute the following c code?
int * call();
void main(){
int *ptr;
ptr=call();
clrscr();
printf("%d",*ptr);
}
int * call(){
int a=25;
a++;
return &a;
}
What is error in following declaration?
struct outer{
int a;
struct inner{
char c;
};
};
What will be output if you will compile and execute the following c code?
void main(){
int array[]={10,20,30,40};
printf("%d",-2[array]);
}
What will be output if you will compile and execute the following c code?
void main(){
int i=10;
static int x=i;
if(x==i)
printf("Equal");
else if(x>i)
printf("Greater than");
else
printf("Less than");
}
What will be output if you will compile and execute the following c code?
#define max 5;
void main(){
int i=0;
i=max++;
printf("%d",i++);
}
What will be output if you will compile and execute the following c code?
void main(){
double far* p,q;
printf("%d",sizeof(p)+sizeof q);
}
15 comments:
Really Its awesome.....
Answer of Question number is=19
How? not 21 why ?
19.
What will be output if you will compile and execute the following c code?
#define max 5;
void main(){
int i=0;
i=max++;
printf("%d",i++);
}
The correct answer is :
(E) Compiler error
Explanation:
file: test.i
test.c 1:
test.c 2: void main(){
test.c 3: int i=0;
test.c 4: i=5;++; //because u have define max as 5;
test.c 5: printf("%d",i++);
test.c 6: }
test.c 7:
C:\Users\Robert\Documents\c\test9\56789.c||In function 'main':|
C:\Users\Robert\Documents\c\test9\56789.c|4|error: expected expression before ';' token|
||=== Build finished: 1 errors, 0 warnings ===|
gud work....
This code gives me compiler error
#define max 5;
void main(){
int i=0;
i=max++;
printf("%d",i++);
}
/////////////////////
The output of this code is 19 and I don't know why !!
void main(){
int i=4,x;
x=++i + ++i + ++i;
printf("%d",x);
}
NYC...questions...plzzz increases the no of questions
Very nice questions,beautifully explained.
Thanks a lot brother.
very nice questions...plzzz increases the no of questions
whether we can use double quotes to include header files
All questions. are very good but..less no of questions
yes kushi chopra you can include but there are two ways... just presentation is different
1) ==> system define .h(header file)
2) "myhead.h" ==> user define .h(header file)
its really nice c test skills............
it depends upon compiler used..
for gcc its 21
for turbo its 19
Great Website.
its true
Post a Comment